Merge Link
Merge Link is a UI component that guides your app's user through setting up an integration.
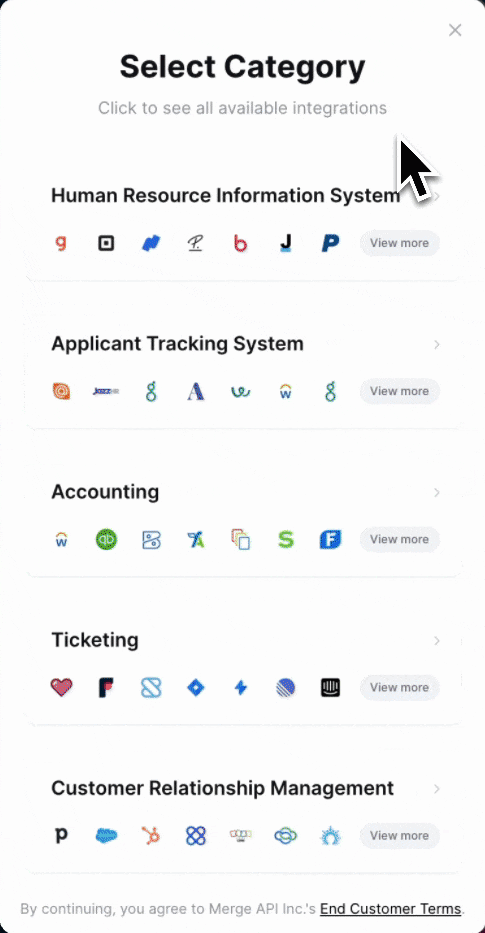
Add Merge Link to Your Product
Merge Link utilizes a series of token exchanges to securely authenticate your users' integrations.
In this guide, you'll set up the following in your application:
- Get a
link_token
to initialize a Merge Link session for your end user. - Make Merge Link appear in your frontend.
- Swap for an
account_token
, which authenticates future requests to the Unified API.
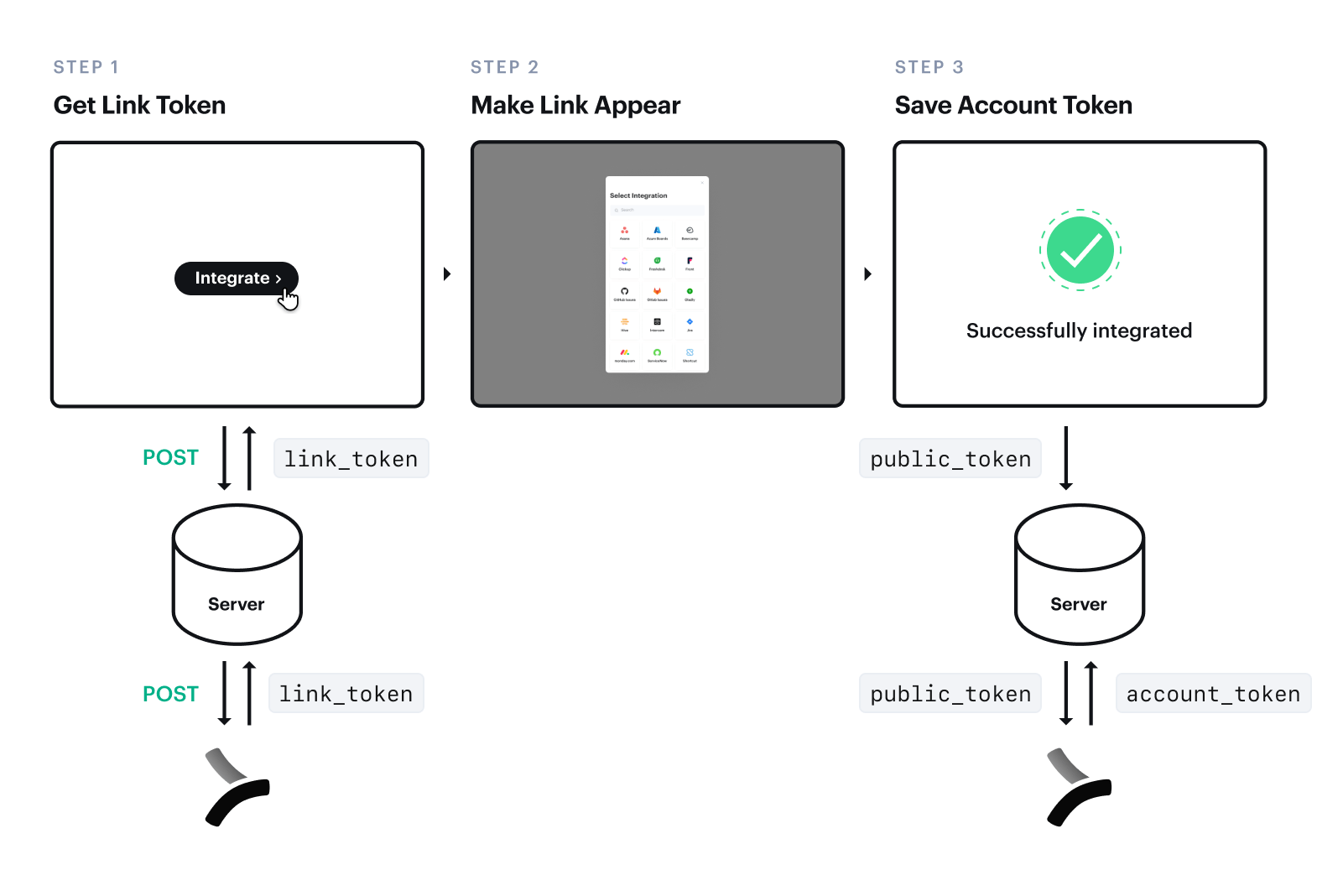
Backend – Get Link Token
In your backend, set up a POST request to initialize a Merge Link session and get a link_token
from this URL:
https://api.merge.dev/api/integrations/create-link-token
Note: If you are using our SDKs, you can get a Link Token using a category-specific endpoint. For example, for HRIS, it can be found at https://docs.merge.dev/hris/link-token.
Pass in your Production Access API key as a header.
Configure your Merge Link with the following parameters:
Parameter | Type | Description |
end_user_origin_id | String | Unique ID for your end user. For more information see End User Origin ID guide. |
end_user_organization_name | String | Your end user's organization. |
end_user_email_address | String | Your end user's email address. |
categories | Array | The integration categories to show in Merge Link.["hris", "ats", "accounting", "ticketing", "crm", "mktg", "filestorage"] |
integration Optional | String | Identifier of third-party platform to skip Merge Link menu for. See Single Integration guide. |
link_expiry_mins Optional | Integer | An integer number of minutes between [30, 720 or 10080 if for a Magic Link URL] for how long this token is valid. Defaults to 30. |
should_create_magic_link_url Optional | Boolean | Whether to generate a Magic Link URL. Defaults to false. For more information on Magic Link, see Magic Link guide. |
The response will include the following fields:
Parameter | Type | Description |
link_token | String | Temporary token to initialize your end user's Merge Link. |
integration_name | String | The name of any previously connected third-party platform (otherwise null ). |
magic_link_url | String | The URL of the magic link if specified (otherwise null ). |
Pass the link_token
to your frontend to display Merge Link in step 2.
Each end_user_origin_id
can have a maximum of one Linked Account per category.
For example, each ID can have up to one HR, Payroll, and Directory, one ATS, one Accounting, one Ticketing, one CRM, one Marketing Automation, and one File Storage integration simultaneously.
If you want to link multiple accounts for the same user, learn more in our help center.
Frontend – Make Merge Link Appear
In your frontend, use the link_token
from step 1 to open Merge Link.
Pass in these parameters:
Parameter | Type | Description |
linkToken | String | Initializing token from step 1. |
onSuccess | Function | Callback to handle public_token , which is returned when your end user finishes their Merge Link session. Use it immediately to swap for your account token. |
onExit Optional | Function | Callback to handle when your end user closes Merge Link. You can add your own logic here to define any functionality. |
tenantConfig Optional | Object | Parameter to specify an apiBaseURL for a tenant. For example, for the EU multi-tenant, apiBaseURL should be set to https://api-eu.merge.dev . The default value is https://api.merge.dev . |
Pass the public_token
to your backend to securely swap it for an account_token
in step 3.
Backend – Swap public token for account token
In your backend, create a request to exchange the short-lived public_token
for a permanent account_token
.
Important: Securely store this account_token
in your database for authenticating future API requests to the Unified API regarding the end user's data.
Discover other Merge features in our Guides or learn more about Unified API functionality in the links below.